Laravel has a powerful set of validation rules, but sometimes you need to validate the specific types of files and not all scenarios are explained in Laravel documentation. In this article, I will observe the ways to validate different files such as images, base64, audio and video, CSV, Excel files, and others. Please choose which type of file you need to validate in the table of contents below.
Table of Contents
- Main file validators
- How to validate multiple files in Laravel?
- How to validate images in Laravel?
- How to validate base64 files Laravel?
- How to validate audio and video files in Laravel?
- How to validate CSV files in Laravel?
- How to validate Excel files in Laravel?
- How to validate XML files in Laravel?
Main file validators
To validate successfully uploaded file You can use file validator:
'file_input' => 'file',
To make file input required You can use the required validator same as with any other input types:
'file_input' => 'file|required',
MIME type validators
To validate the MIME type of uploaded file use the mimetypes validator:
'file_input' => 'file|mimetypes:text/plain,text/html',
To validate the MIME type of uploaded file by file extension use mimes validator:
'file_input' => 'file|mimes:jpg,png',
File size validators
If You want to validate the file against the exact file size in KB then use the size validator:
'file_input' => 'file|size:1024',
Use max validator to check if the file size is not greater than the given value:
'file_input' => 'file|max:1024',
Use min validator to check if the file size is not less than the given value:
'file_input' => 'file|min:1024',
Or You can use between validator to check if the file size is between given min and max values:
'file_input' => 'file|between:1024,2048',
How to validate multiple files in Laravel?
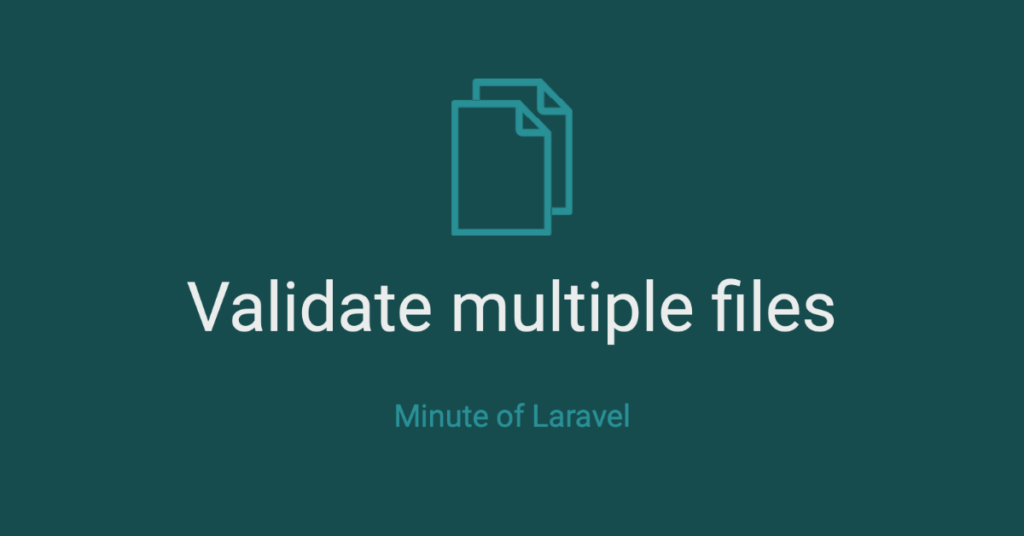
If you need to validate an array of files in Laravel then your input should have the multiple attribute and the input name should be an array, like in the example below:
<input type="file" id="file" name="user_files[]" multiple>
And then add dot following with asterisk symbol near input name in your validation rules:
'user_files.*' => 'file|between:1024,2048',
If for some reason you need to validate each uploaded file from array differently then you can specify a specific index of file:
'user_files.1' => 'file|between:1024,2048',
'user_files.2' => 'file|between:2048,4096',
Note that if you want to make multiple files input to be required then you should not use dot/asterisk or dot/index:
'user_files' => 'required',
'user_files.*' => 'file|between:1024,2048',
How to validate images in Laravel?
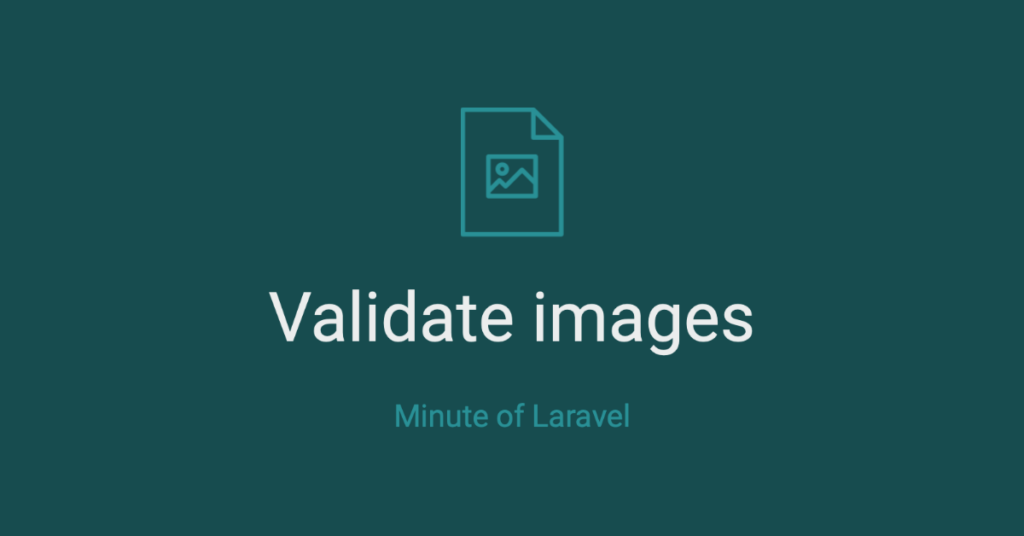
To check if the uploaded file is an image with then use the image validator. This validator checks if the file has one of these extensions: jpg, jpeg, png, bmp, gif, svg, or webp.
'file_input' => 'image',
If you need to validate an image against custom MIME types then use MIME type validators. If you need to validate the image file size then use file size validators. These validators are explained above. An example of validating an image against custom MIME types and file size could be:
'file_input' => 'file|mimes:jpg,png|max:512',
How to validate image dimensions?
To validate image dimensions Laravel has the dimensions validator which can be used with 7 constraints:
- min_width
- max_width
- min_height
- max_height
- width
- height
- ratio
Example of validating image dimensions:
'image' => 'dimensions:max_width=1000,max_height=500,ratio=2/1',
How to validate base64 files in Laravel?
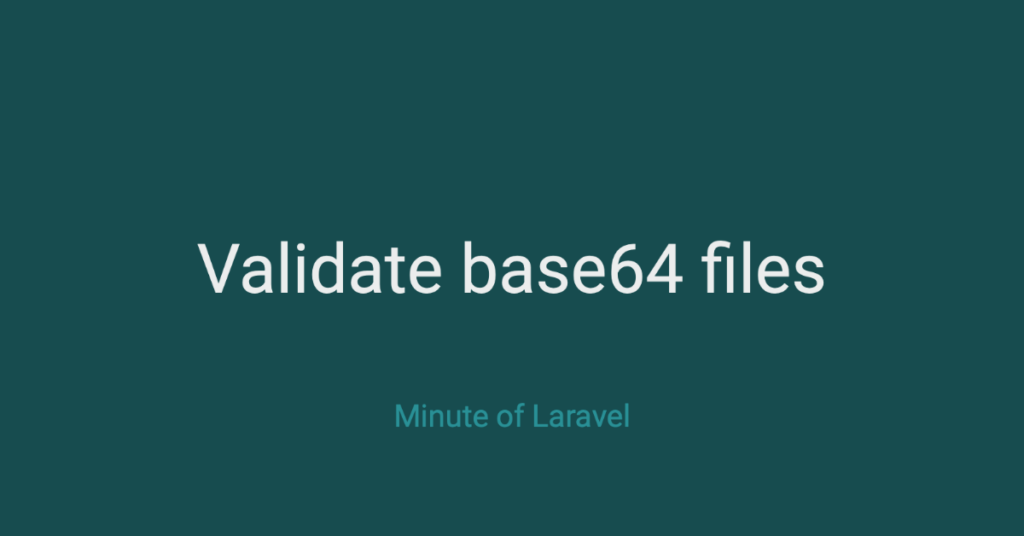
Sometimes you need to validate base64 files. For example, in the frontend, you have an image creation tool that sends created images in base64 format to the backend. Of course, you should validate this base64 encoded image for security reasons, and also maybe you need to validate base64 image dimensions or so. In this case, I would recommend the crazybooot/base64-validation package for Laravel.
It is easy to install with composer:
composer require crazybooot/base64-validation
The package has these validators:
- base64max
- base64min
- base64dimensions
- base64file
- base64image
- base64mimetypes
- base64mimes
- base64between
- base64size
These validators are equivalent to the native Laravel file validators I wrote about above. There is an example of how to use them:
'created_image' => 'base64image|base64size:1024|base64dimensions:min_width=100,min_height=200',
This package uses error messages from its equivalent from the native Laravel file validator. For example, the base64image validator will show an error message of the native Laravel image validator. If you want to use your own error messages you need to publish package configuration file with this command:
php artisan vendor:publish --provider="Crazybooot\Base64Validation\Providers\ServiceProvider" --tag=config
This command will add config/base64validation.php file. Open it and change replace_validation_message to false:
'replace_validation_messages' => false,
And then just add validation error messages as you simply add them. For example, open the resources/lang/en/validation.php file and add these lines:
'base64max' => 'Your custom error message for base64 validator',
'base64min' => 'Your custom error message for base64 validator',
'base64dimensions' => 'Your custom error message for base64 validator',
'base64file' => 'Your custom error message for base64 validator',
'base64image' => 'Your custom error message for base64 validator',
'base64mimetypes' => 'Your custom error message for base64 validator',
'base64mimes' => 'Your custom error message for base64 validator',
'base64between' => 'Your custom error message for base64 validator',
'base64size' => 'Your custom error message for base64 validator',
How to validate audio and video files in Laravel?
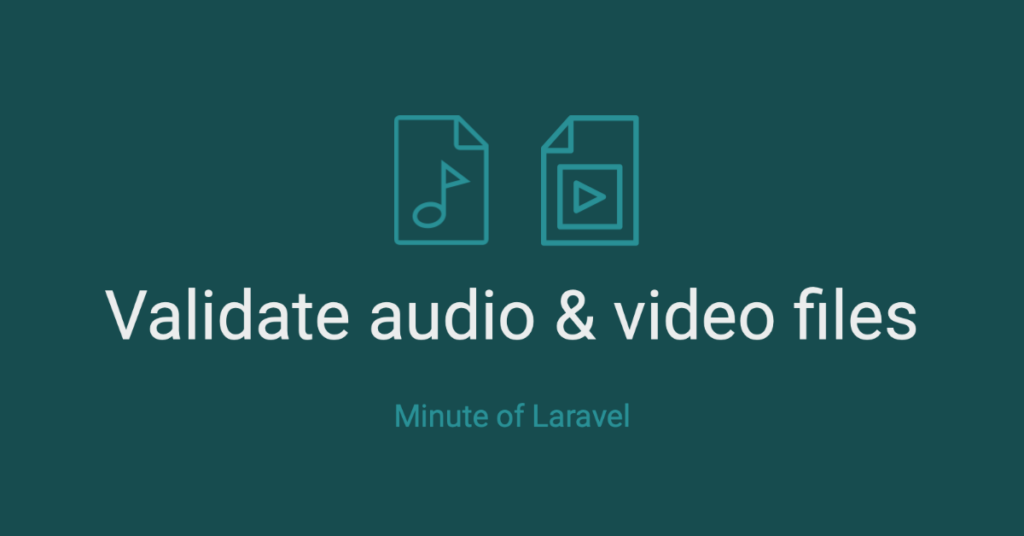
To check if the uploaded file is an audio or video file then use MIME type validators. Below is a list of audio mime types and a list of video mime types. However, Laravel’s video/audio files MIME types guessing is not reliable. That’s because I created a package that adds audio and video validators to your Laravel project which is called minuteoflaravel/laravel-audio-video-validator. To install this package you should install ffmpeg multimedia framework:
- On Debian/Ubuntu, run
sudo apt install ffmpeg
- On macOS with Homebrew:
brew install ffmpeg
After that install the package via composer:
composer require minuteoflaravel/laravel-audio-video-validator
The package adds these validators:
- audio
- video
- codec
- duration
- duration_max
- duration_min
- video_width
- video_height
- video_max_width
- video_max_height
- video_min_width
- video_min_height
Here are examples of how to use those validators:
$request->validate([
'audio' => 'required|audio|duration_min:30|duration_max:300|codec:mp3,pcm_s16le',
]);
$request->validate([
'video' => 'required|video|duration:60|codec:h264|video_width_max:1920|video_height_max:1080',
]);
The first one check if uploaded file is an audio file, if its duration is between 30 and 300 seconds and if an audio file codec name is mp3 or pcm_s16le(.wav). The second one checks if uploaded file is a video file, if its duration is exactly 60 seconds, if its codec name is h264(.mp4) and if its resolution is not greater than 1900×1080.
Audio MIME types list
.aif | audio/aiff |
.aif | audio/x-aiff |
.aifc | audio/aiff |
.aifc | audio/x-aiff |
.aiff | audio/aiff |
.aiff | audio/x-aiff |
.au | audio/basic |
.au | audio/x-au |
.funk | audio/make |
.gsd | audio/x-gsm |
.gsm | audio/x-gsm |
.it | audio/it |
.jam | audio/x-jam |
.kar | audio/midi |
.la | audio/nspaudio |
.la | audio/x-nspaudio |
.lam | audio/x-liveaudio |
.lma | audio/nspaudio |
.lma | audio/x-nspaudio |
.m2a | audio/mpeg |
.m3u | audio/x-mpequrl |
.mid | audio/midi |
.mid | audio/x-mid |
.mid | audio/x-midi |
.midi | audio/midi |
.midi | audio/x-mid |
.midi | audio/x-midi |
.mjf | audio/x-vnd.audioexplosion.mjuicemediafile |
.mod | audio/mod |
.mod | audio/x-mod |
.mp2 | audio/mpeg |
.mp2 | audio/x-mpeg |
.mp3 | audio/mpeg3 |
.mp3 | audio/x-mpeg-3 |
.mpa | audio/mpeg |
.mpg | audio/mpeg |
.mpga | audio/mpeg |
.my | audio/make |
.pfunk | audio/make |
.pfunk | audio/make.my.funk |
.qcp | audio/vnd.qcelp |
.ra | audio/x-pn-realaudio |
.ra | audio/x-pn-realaudio-plugin |
.ra | audio/x-realaudio |
.ram | audio/x-pn-realaudio |
.rm | audio/x-pn-realaudio |
.rmi | audio/mid |
.rmm | audio/x-pn-realaudio |
.rmp | audio/x-pn-realaudio |
.rmp | audio/x-pn-realaudio-plugin |
.rpm | audio/x-pn-realaudio-plugin |
.s3m | audio/s3m |
.sid | audio/x-psid |
.snd | audio/basic |
.snd | audio/x-adpcm |
.tsi | audio/tsp-audio |
.tsp | audio/tsplayer |
.voc | audio/voc |
.voc | audio/x-voc |
.vox | audio/voxware |
.vqe | audio/x-twinvq-plugin |
.vqf | audio/x-twinvq |
.vql | audio/x-twinvq-plugin |
.wav | audio/wav |
.wav | audio/x-wav |
.xm | audio/xm |
Video MIME types list
.afl | video/animaflex |
.asf | video/x-ms-asf |
.asx | video/x-ms-asf |
.asx | video/x-ms-asf-plugin |
.avi | video/avi |
.avi | video/msvideo |
.avi | video/x-msvideo |
.avs | video/avs-video |
.dif | video/x-dv |
.dl | video/dl |
.dl | video/x-dl |
.dv | video/x-dv |
.fli | video/fli |
.fli | video/x-fli |
.fmf | video/x-atomic3d-feature |
.gl | video/gl |
.gl | video/x-gl |
.isu | video/x-isvideo |
.m1v | video/mpeg |
.m2v | video/mpeg |
.mjpg | video/x-motion-jpeg |
.moov | video/quicktime |
.mov | video/quicktime |
.movie | video/x-sgi-movie |
.mp2 | video/mpeg |
.mp2 | video/x-mpeg |
.mp2 | video/x-mpeq2a |
.mp3 | video/mpeg |
.mp3 | video/x-mpeg |
.mpa | video/mpeg |
.mpe | video/mpeg |
.mpeg | video/mpeg |
.mpg | video/mpeg |
.mv | video/x-sgi-movie |
.qt | video/quicktime |
.qtc | video/x-qtc |
.rv | video/vnd.rn-realvideo |
.scm | video/x-scm |
.vdo | video/vdo |
.viv | video/vivo |
.viv | video/vnd.vivo |
.vivo | video/vivo |
.vivo | video/vnd.vivo |
.vos | video/vosaic |
.xdr | video/x-amt-demorun |
.xsr | video/x-amt-showrun |
How to validate CSV files in Laravel?
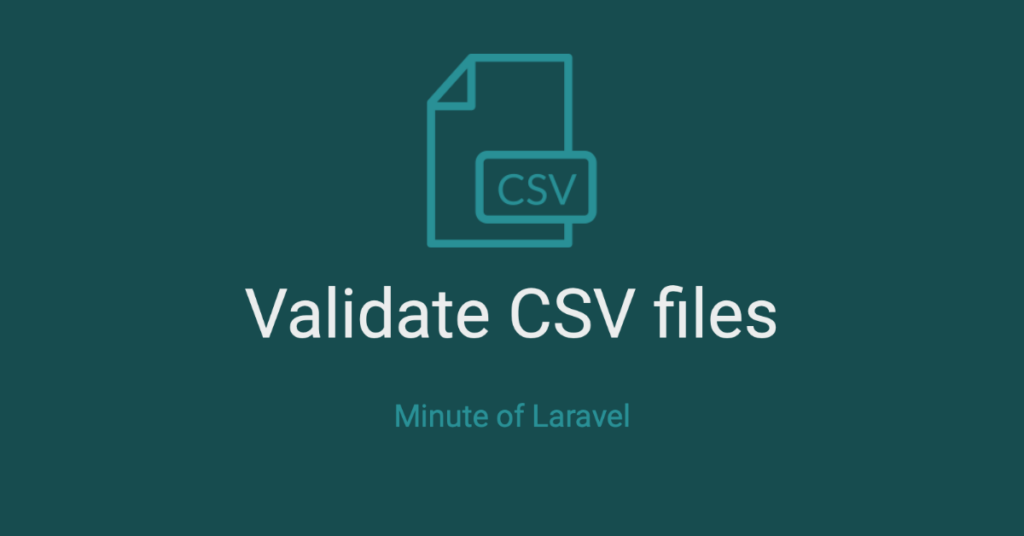
When we are talking about CSV files validation then we mean one of three different validation variants:
- Check if MIME type or file extention matches your needs
- Check if uploaded file is formatted in CSV format
- Check if uploaded file CSV file structure matches yout needs
The first variant should be used when you want to check if the uploaded file is a CSV file. To do this use the MIME type or extension validators but always remember that the user can rename any file extension to *.csv and also if you validate against MIME type then keep in mind that a real CSV file can have the text/plain MIME type. So in my opinion, if you need to be sure that the uploaded file is a CSV file then you should use the second validation variant where you check if the uploaded file is formatted in CSV format. You should use a third validation variant when you want to validate cells of the uploaded CSV file. Below I will review the second and third variants.
To check if the uploaded file is formatted in CSV format then you can use the simple package that I created for this purpose which is called minuteoflaravel/laravel-csv-validator. CSV validator, which is added by this package, parses the uploaded file using parsecsv/php-parsecsv library and if there are no errors during file parsing then validation is passed. Install this package with composer:
composer require minuteoflaravel/laravel-csv-validator
And then add csv validator to your validation rules:
'uploaded_file' => 'csv',
You can customize the error message. Open resources/lang/en/validation.php file and add your message:
'csv' => 'The :attribute must be a CSV file.',
If you choose to validate with a third validation variant – CSV file cells validation – then I suggest using SUKOHI/CsvValidator package. This package allows you to select default Laravel validation rules for each cell. To install this package run the composer command:
composer require sukohi/csv-validator:3.*
Then add validation rules for columns to array:
$csv_rules = [
0 => 'required',
1 => 'integer',
2 => 'required|min:10'
];
Create options array:
$options = [
'encoding' => 'sjsin-win',
'start_row' => 1, // <- Starting validation from row one, not zero.
'end_row' => 4, // <- Ending validation
'row_callback' => function($row_number, $row_data) {
return true; // `false` means skipping validation
}
];
And then add a validator to your validation rules:
$request->validate([
'csv_file' => [
new Csv($csv_rules, $options)
]
]);
If you need to add validation rules for cells by column name then I suggest using another package which is called Konafets/laravel-csv-validator. This package is similar to the previous one but by using it you can add a validation rule to columns by column name from header row. To install:
composer require konafets/laravel-csv-validator:1.0-dev
And there is a code example of how to use this package:
$csvPath = 'employees.csv';
$rules = [
'First Name' => 'required|string',
'Last Name' => 'required|string',
'Year of Birth' => 'required|numeric',
];
$csvValidator = CsvValidator::make($csvPath, $rules);
if($csvValidator->fails()) {
$errors = $csvValidator->getErrors();
} else {
$csvData = $csvValidator->getData();
}
How to validate Excel files in Laravel?
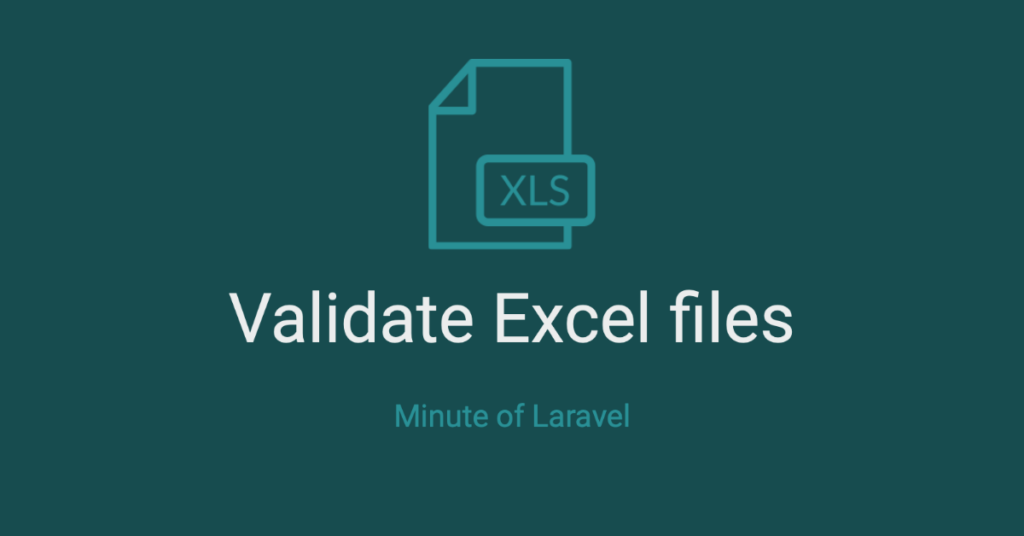
To validate Excel files you can use MIME type validators. Here is an example of how to validate against few MIME types:
'file_input' => 'file|mimetypes:application/vnd.openxmlformats-officedocument.spreadsheetml.sheet,application/excel',
In the example added only two MIME types. Below is full list of Excel MIME types.
Excel MIME types list
.xlsx | application/vnd.openxmlformats-officedocument.spreadsheetml.sheet |
.xlsm | application/vnd.ms-excel.sheet.macroEnabled.12 |
xlsb | application/vnd.ms-excel.sheet.binary.macroEnabled.12 |
.xl | application/excel |
.xla | application/excel |
.xla | application/x-excel |
.xlb | application/excel |
.xlb | application/vnd.ms-excel |
.xlb | application/x-excel |
.xlc | application/excel |
.xlc | application/vnd.ms-excel |
.xlc | application/x-excel |
.xld | application/excel |
.xld | application/x-excel |
.xlk | application/excel |
.xlk | application/x-excel |
.xll | application/excel |
.xll | application/vnd.ms-excel |
.xll | application/x-excel |
.xlm | application/excel |
.xlm | application/vnd.ms-excel |
.xlm | application/x-excel |
.xls | application/excel |
.xls | application/vnd.ms-excel |
.xls | application/x-msexcel |
.xls | application/x-excel |
.xlt | application/excel |
.xlt | application/x-excel |
.xlv | application/excel |
.xlv | application/x-excel |
.xlw | application/excel |
.xlw | application/vnd.ms-excel |
.xlw | application/x-excel |
How to validate XML files in Laravel?
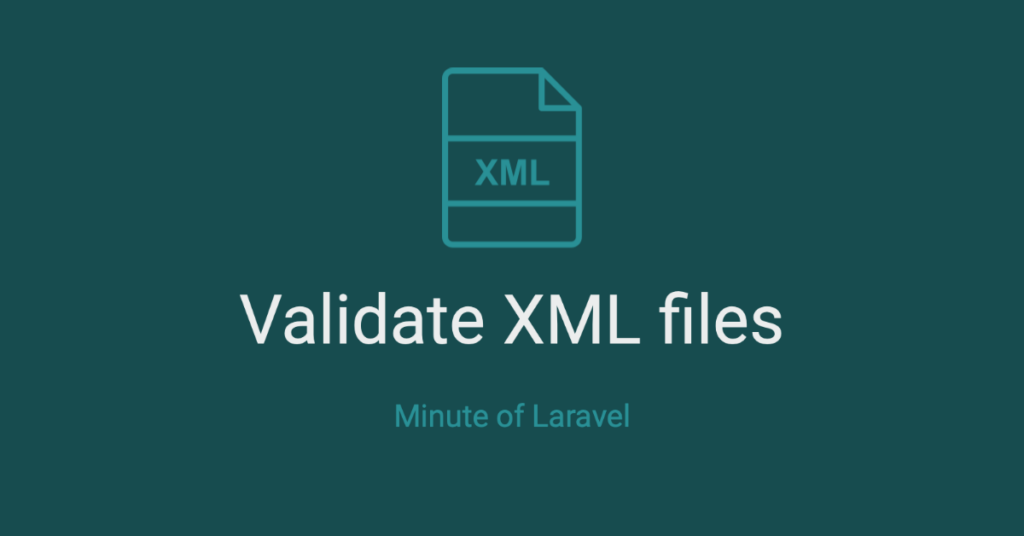
To validate XML files you can use MIME type validators. Here is an example:
'file_input' => 'file|mimetypes:application/xml,text/xml',